Kubernetes Health Check
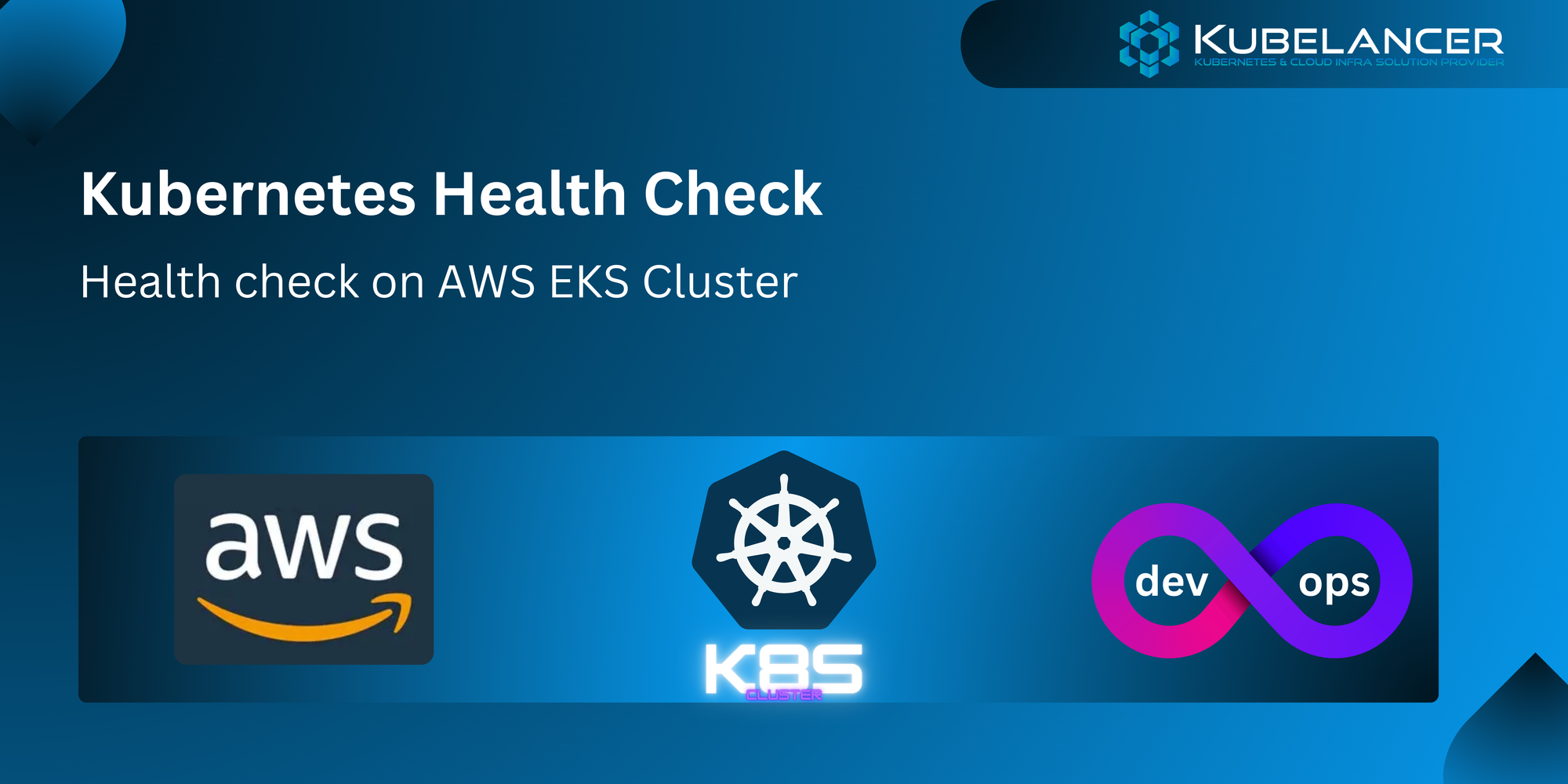
Kubernetes health check refers to a mechanism used to monitor the health of individual containers and services for detecting issues proactively and ensuring that applications are running smoothly and are available to handle requests.
The three types of Kubernetes health checks are:
- Liveness Probe - This check determines whether the application is running correctly.
- Readiness Probe - This check determines whether the application is ready to serve traffic.
- Startup Probe - Startup probes are used to determine when a container application has been initialized successfully. If a startup probe fails, the pod is restarted.
Kubernetes supports various protocols for health checks, each with its own benefits and limitations. Selecting the appropriate protocol based on your use case can help ensure the reliability and availability of your applications.
Some of the protocols are ,
- HTTP
- HTTPS
- TCP
- Command probes
- gRPC
Configure Probes
initialDelaySeconds
: Number of seconds after the container has started before startup, liveness or readiness probes are initiated. If a startup probe is defined, liveness and readiness probe delays do not begin until the startup probe has succeeded. If the value ofperiodSeconds
is greater thaninitialDelaySeconds
then theinitialDelaySeconds
would be ignored. Defaults to 0 seconds. Minimum value is 0.periodSeconds
: How often (in seconds) to perform the probe. Default to 10 seconds. The minimum value is 1.timeoutSeconds
: Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1.successThreshold
: Minimum consecutive successes for the probe to be considered successful after having failed. Defaults to 1. Must be 1 for liveness and startup Probes. Minimum value is 1.failureThreshold
: After a probe failsfailureThreshold
times in a row, Kubernetes considers that the overall check has failed: the container is not ready/healthy/live. For the case of a startup or liveness probe, if at leastfailureThreshold
probes have failed, Kubernetes treats the container as unhealthy and triggers a restart for that specific container. The kubelet honors the setting ofterminationGracePeriodSeconds
for that container. For a failed readiness probe, the kubelet continues running the container that failed checks, and also continues to run more probes; because the check failed, the kubelet sets theReady
condition on the Pod tofalse
.terminationGracePeriodSeconds
: configure a grace period for the kubelet to wait between triggering a shut down of the failed container, and then forcing the container runtime to stop that container. The default is to inherit the Pod-level value forterminationGracePeriodSeconds
(30 seconds if not specified), and the minimum value is 1. See probe-levelterminationGracePeriodSeconds
for more detail.
Demo:
Create simple Go app
vi main.go
package main
import (
"fmt"
"log"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "App Demo: Hello, World!")
}
func healthCheckHandler(w http.ResponseWriter, r *http.Request) {
w.WriteHeader(http.StatusOK)
fmt.Fprintf(w, "App health: Health Check OK")
}
func main() {
http.HandleFunc("/", handler)
http.HandleFunc("/health", healthCheckHandler)
fmt.Println("Starting server on port 8080...")
log.Fatal(http.ListenAndServe(":8080", nil))
}
Build and Push Docker image to Dockerhub
docker buildx build --push --platform linux/arm64,linux/amd64 --tag hariharankube/heathcheck:v1 .
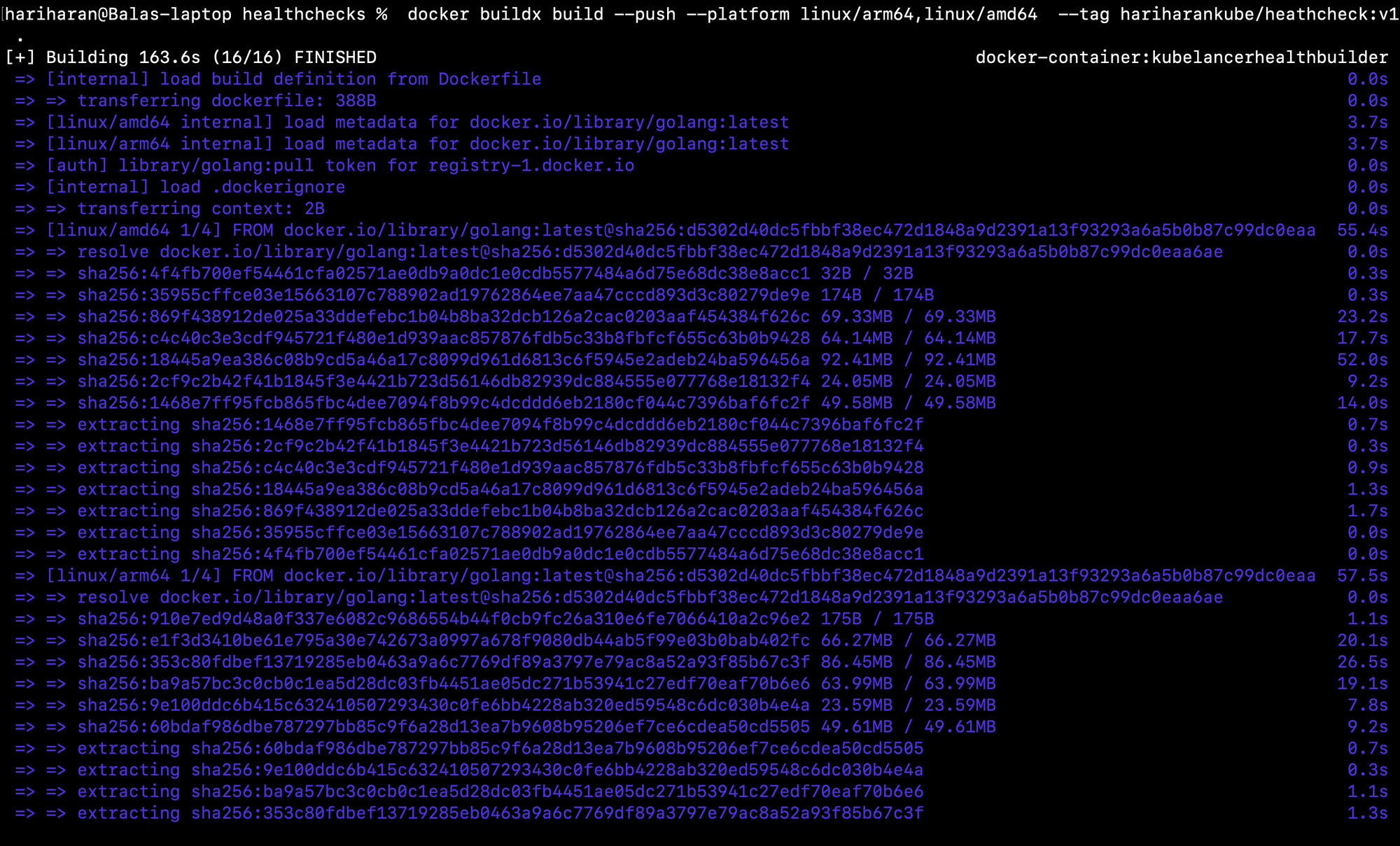
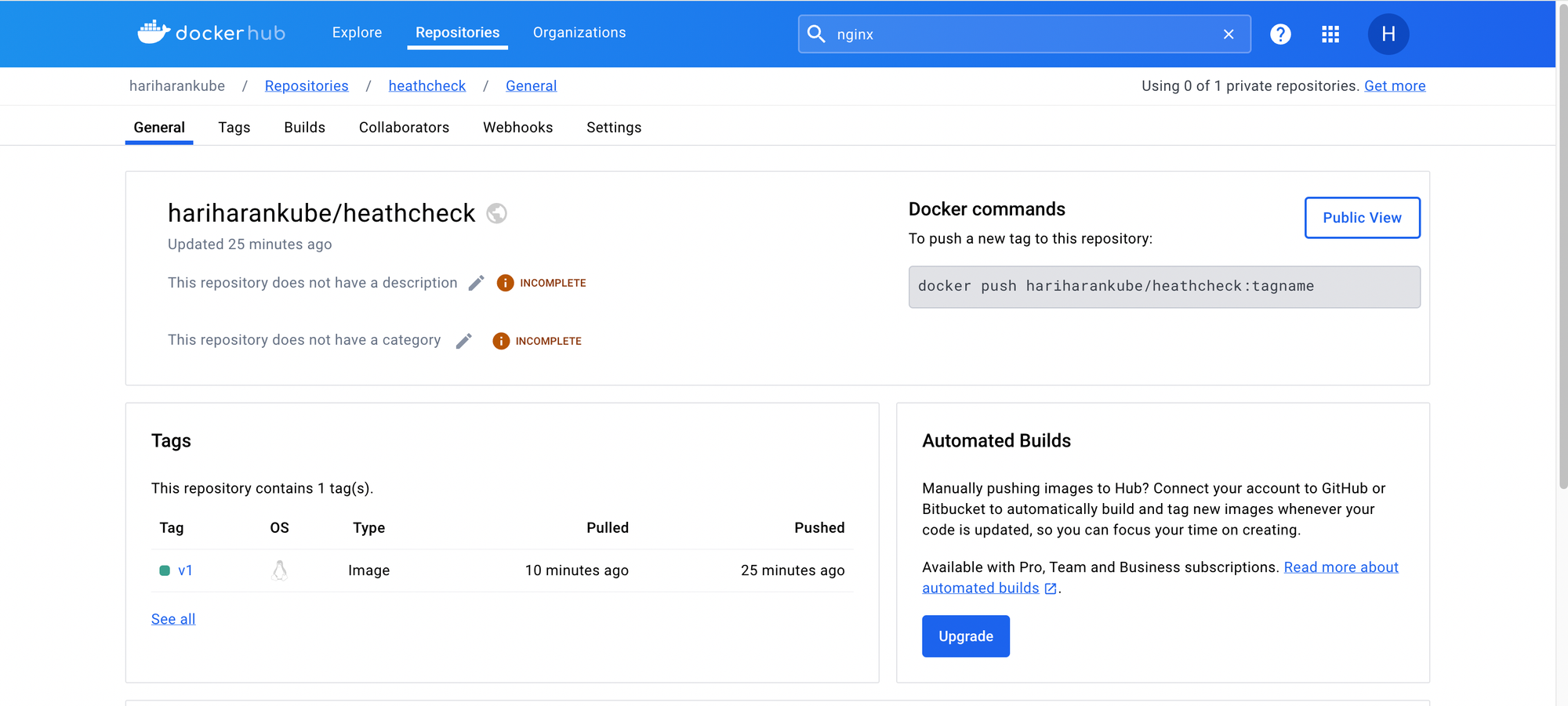
Sample Deployments
Liveness Probe
Using HTTP protocol for Kubernetes health check
apiVersion: v1
kind: Pod
metadata:
name: live-http
spec:
containers:
- name: live-container
image: hariharankube/heathcheck:v1
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 5
periodSeconds: 3
kubectl get pod

kubectl describe po live-http

Readiness probes are configured similarly to liveness probes
Readiness Probe
Using HTTP protocol for Kubernetes health check
apiVersion: v1
kind: Pod
metadata:
name: read-http
spec:
containers:
- name: read-container
image: hariharankube/heathcheck:v1
readinessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 5
periodSeconds: 3
kubectl get pod read-http

kubectl describe pod read-http

Startup Probe
Startup probe allows our application to become ready, joined with readiness and liveness probes, it can dramatically increase our applications' availability.
Sample Nginx Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: startup-probes
labels:
app: nginx
spec:
replicas: 1
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: hariharankube/heathcheck:v1
ports:
- containerPort: 80
startupProbe:
initialDelaySeconds: 1
periodSeconds: 2
timeoutSeconds: 1
successThreshold: 1
failureThreshold: 1
exec:
command:
- /health
apiVersion: v1
kind: Service
metadata:
labels:
app: nginx
name: startup-probe
namespace: default
spec:
ports:
- name: nginx-http-port
port: 80
selector:
app: nginx
sessionAffinity: None
type: NodePort
kubectl get deploy
kubectl get po
kubectl get svc
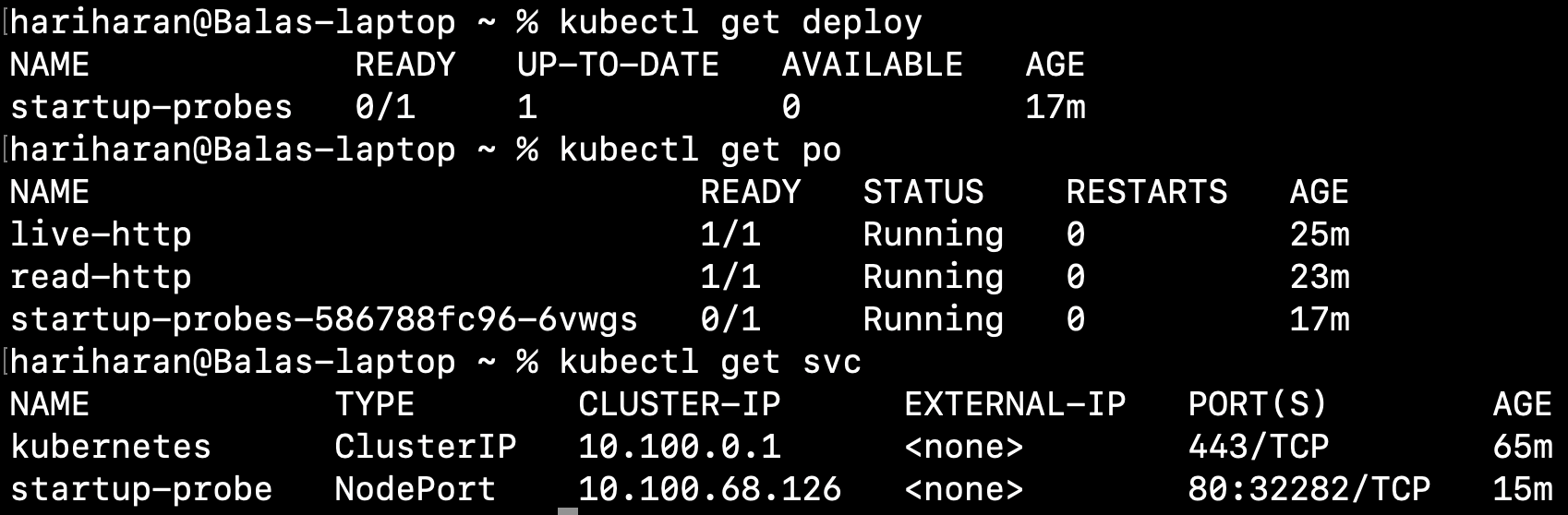
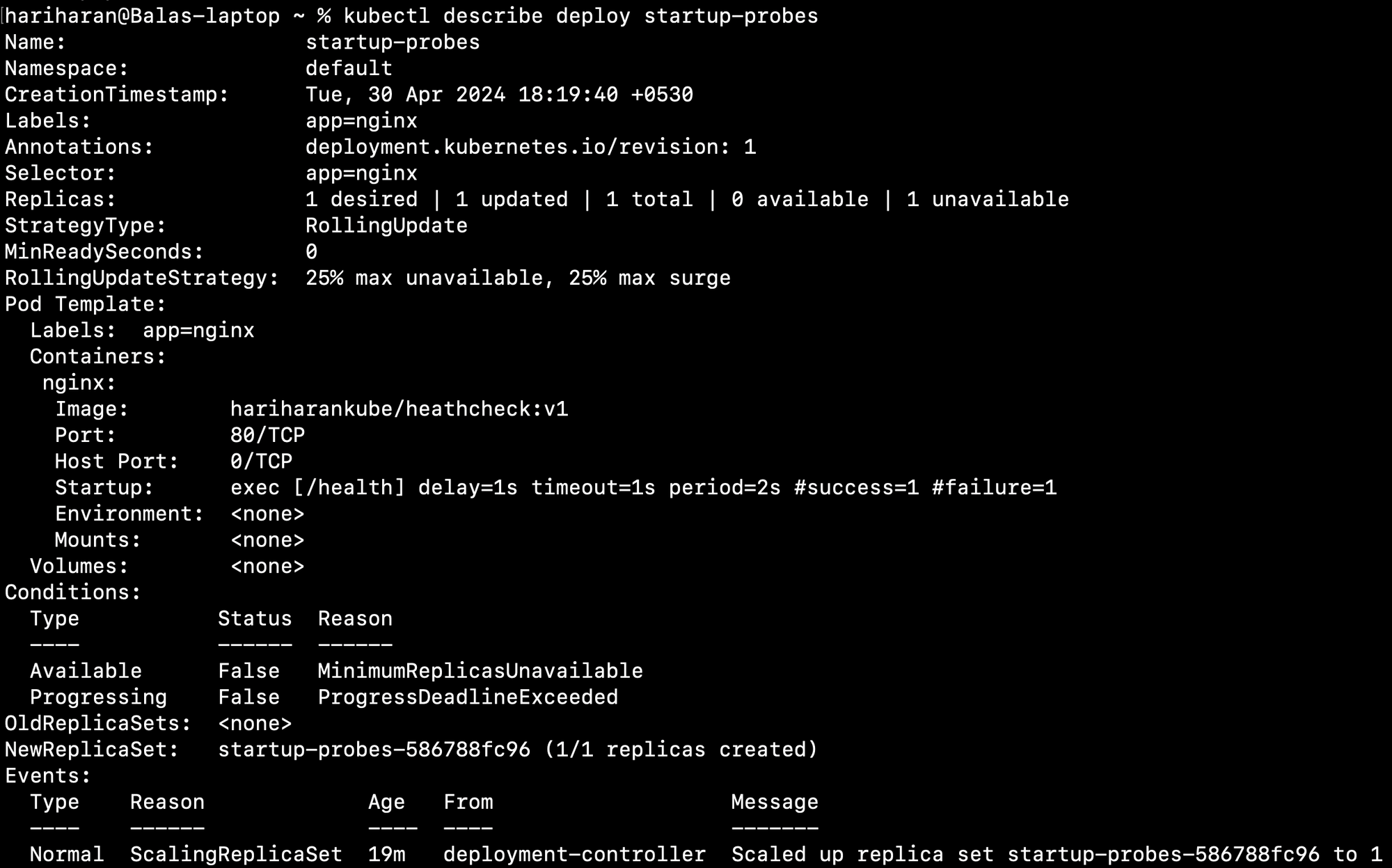